Custom Mouse Cursor is here for your website.
what makes a website interesting are its small creative features and one of them could be a Custom Mouse Cursor. We use mouse cursor to basically navigate every where in the website. So what if we can make it better and eye capturing for our viewers.
It is possible that viewers may recall a particular feature that you have implemented on your website. Some may appreciate it and revisit the website simply for the pleasure of experiencing it again. Others may view this as the work put into modifying even the smallest detail on the website and be curious how they can do it . Here is a Custom Cursor that you can use to learn and implement on your website.
Basic Knowledge necessary-
This blog/article is designed for beginners, but to fully benefit from it, it would be helpful to have a basic understanding of the following concepts:
- HTML
- Basic CSS
- Basic JavaScript
What is a Custom Cursor?
Instead of boring pointy cursor that we see everyday a custom cursor is an intentional change made to the default icon that shows you where your mouse is pointing or hovering.
It’s really easy to change the default cursor on a computer through the settings. For a webpage, you just need a little HTML, CSS, and JavaScript. Don’t be intimidated, it’s really simple!
How to make Custom Mouse cursor using HTML, CSS and JavaScript?
To make a custom cursor for your webpage we first need to open you a code editor . Let’s open up VS-Code in your windows or in mac. You can use any other code editors if you prefer them. Open up any folder inside the code editor.
Let’s create a html, CSS and aa JavaScript file inside a folder and get ready to write some code.
HTML Code-
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<link rel="stylesheet" href="style.css">
<title>Custom Cursor</title>
</head>
<body>
<div id="main">
<div class="cursor"></div>
<h1>Codeallready</h1>
</div>
<script src="script.js"></script>
</body>
</html>
Here we have created an id named “main”. Inside the main id there are two Html tags , a div
with the class named “cursor” and a H1 tag
.
We have linked our CSS with a link tag- <link rel="stylesheet" href="style.css">
To make it more stylish here the CSS code-
CSS Code-
@import url('https://fonts.googleapis.com/css2?family=DM+Sans:ital,opsz,wght@0,9..40,100..1000;1,9..40,100..1000&family=Roboto:ital,wght@0,100;0,300;0,400;0,500;0,700;0,900;1,100;1,300;1,400;1,500;1,700;1,900&display=swap');
* {
margin: 0;
padding: 0;
box-sizing: border-box;
text-decoration: none;
list-style: none;
font-family: "dm sans";
color: whitesmoke;
}
html,
body {
width: 100%;
height: 100%;
}
body {
display: flex;
align-items: center;
justify-content: center;
background-color: rgb(97, 85, 85);
overflow: hidden;
}
#main{
width: 100%;
height: 100%;
background-color: black;
display: flex;
align-items: center;
justify-content: center;
}
.cursor {
height:20px;
width: 20px;
background-color:white;
border-radius: 50%;
position:absolute;
transition: all linear 0.15s;
mix-blend-mode: difference;
}
h1{
font-size: 10vw;
font-weight: 900;
cursor:pointer;
}
This CSS snippet is used to create a fullscreen layout with a customized cursor that changes its appearance when hovering over elements like text. Let’s break it down, with a special focus on the mix-blend-mode: difference
property:
Simple Explanation of the CSS for beginners:
- Global Styles (
*
):- The
*
selector applies styles to all elements. This resets margin, padding, and appliesbox-sizing: border-box
(ensures padding and borders are included in an element’s total width and height). - A font called DM Sans is applied to all text, and the color of the text is set to whitesmoke.
text-decoration: none
removes underlines from links, andlist-style: none
removes bullets from lists.
- The
- HTML and Body Styles:
- The
html
andbody
elements are set to occupy the full width and height of the viewport (width: 100%; height: 100%
). - The
body
is centered both vertically and horizontally usingdisplay: flex
,align-items: center
, andjustify-content: center
. - A background color of
rgb(97, 85, 85)
(darkish brown) is applied to the entire body.
- The
- Main Container (
#main
):- The
#main
container takes up the full viewport (width: 100%; height: 100%
) with a black background. - Like the body, it uses flexbox to center its contents both horizontally and vertically.
- The
- Custom Cursor (
.cursor
):- This is a small, white circular cursor with a height and width of 20px.
- The cursor is positioned absolutely, so it can move freely within the container.
- The
transition: all linear 0.15s
smoothens the movement when the cursor changes position.
h1
Heading:- The
h1
is a large text element (font-size: 10vw
), and its font weight is set to 900, making it bold. - The cursor will change when hovered over this heading (
cursor: pointer
).
- The
mix-blend-mode: difference
Property:
This is the most interesting part of the CSS. The mix-blend-mode
property determines how an element’s content should blend with the background or the content underneath it. Specifically, mix-blend-mode: difference
creates a high-contrast effect by subtracting the color values of the element from the background’s color values. This makes the cursor dynamically change its appearance depending on what’s behind it.
In this case, the white circular cursor is set to blend in “difference mode” with whatever it hovers over. Here’s how it works:
- When the cursor is over white text, it turns black (since white minus white results in black).
- When the cursor is over black or darker backgrounds, it stays white, ensuring visibility.
What is mix-blend-mode
?
The mix-blend-mode
property defines the manner in which an element’s content interacts with the content situated beneath it. This concept bears resemblance to the blending modes observed in graphic design software such as Photoshop, wherein layers interact in order to create visual effects.
Here are some common values for mix-blend-mode
:
- normal: The default. No blending; the element appears as usual.
- multiply: Darkens the background by multiplying the color values.
- screen: Lightens the background by inverting, multiplying, and inverting the colors again.
- overlay: A mix of multiply and screen. It darkens darker areas and lightens lighter areas.
- difference: Subtracts the color values of the background from the element, creating high-contrast effects (as explained earlier).
In this context, using mix-blend-mode: difference
gives a cool interactive effect where the cursor’s color adapts to its background, making it visible on both light and dark areas dynamically.
What has the CSS done ?
So, basically here the CSS creates a full-screen layout where the cursor is styled as a small white circle that blends differently depending on the background it’s hovering over. The mix-blend-mode: difference
ensures the cursor’s visibility by inverting its color based on the background, creating a high-contrast, visually striking effect. This kind of effect is great for enhancing user experience and adding dynamic interactions to a website.
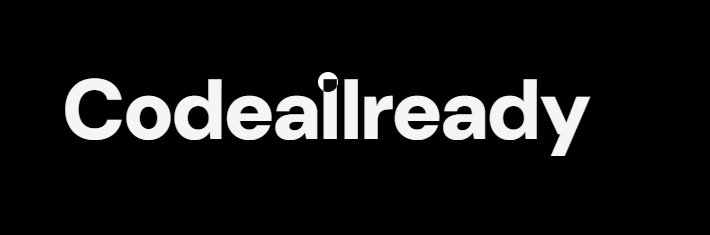
To make it functional here is the JavaScript code
Use JavaScript code to make the Cursor move-
var main = document.querySelector("#main")
var moveCursor = document.querySelector(".cursor");
moveCursor.style.backgroundColor = "white";
main.addEventListener("mousemove",function(mover){
moveCursor.style.left = mover.x+"px"
moveCursor.style.top = mover.y+"px"
})
JavaScript code here is used to control the movement of a custom cursor on the webpage. It follows the mouse pointer around the screen and updates its position in real-time. Here’s a simple breakdown of how it works:
Explanation of the JavaScript Code:
- Variables:
var main = document.querySelector("#main")
: This selects the element with the ID#main
from the HTML document. The#main
element covers the entire page (as per your CSS).var moveCursor = document.querySelector(".cursor")
: This selects the element with the class.cursor
, which is the custom circular cursor.
- Setting the Cursor’s Background Color:
moveCursor.style.backgroundColor = "white";
: This line explicitly sets the background color of the custom cursor to white (though this might already be set in your CSS).
- Event Listener for Mouse Movement:
main.addEventListener("mousemove", function(mover) {...})
: This line adds an event listener to the#main
element. The listener listens for themousemove
event (i.e., whenever the mouse moves inside the#main
element). When the event is triggered, it calls the function inside, passing an objectmover
(short for details) that contains information about the mouse’s position.
- Updating the Cursor’s Position:
moveCursor.style.left = mover.x + "px"
: This sets the left position of the custom cursor to match thex
coordinate of the mouse (mover.x
gives the horizontal position of the mouse). The+ "px"
ensures that the value is a valid CSSleft
property with pixel units.moveCursor.style.top = mover.y + "px"
: Similarly, this sets the top position of the custom cursor to the mouse’sy
coordinate (vertical position).
Basically this script makes the .cursor
element (your custom circular cursor) follow the mouse as it moves within the #main
area. When the mouse moves, the cursor’s position (left
and top
CSS properties) is updated to the current x
and y
coordinates of the mouse.
The result is a dynamic, custom mouse cursor that smoothly tracks your mouse movement, enhancing the user experience.
Conclusion
This is one of the simplest and most widely used custom mouse cursors on websites today! We’ve provided the codes and some helpful explanations to make your coding journey a breeze. We hope you’ve enjoyed learning and implementing this on your website.